I’ve been playing guitar for a while and I’ve been writing my tablatures on papers that I ended up lose somewhere. I always was looking for a simple and easy to use tablature editor without the bloated of software like guitar pro and similar. A plus would be if it will be available online.
So I took it like a challenge and I created this tablature editor.
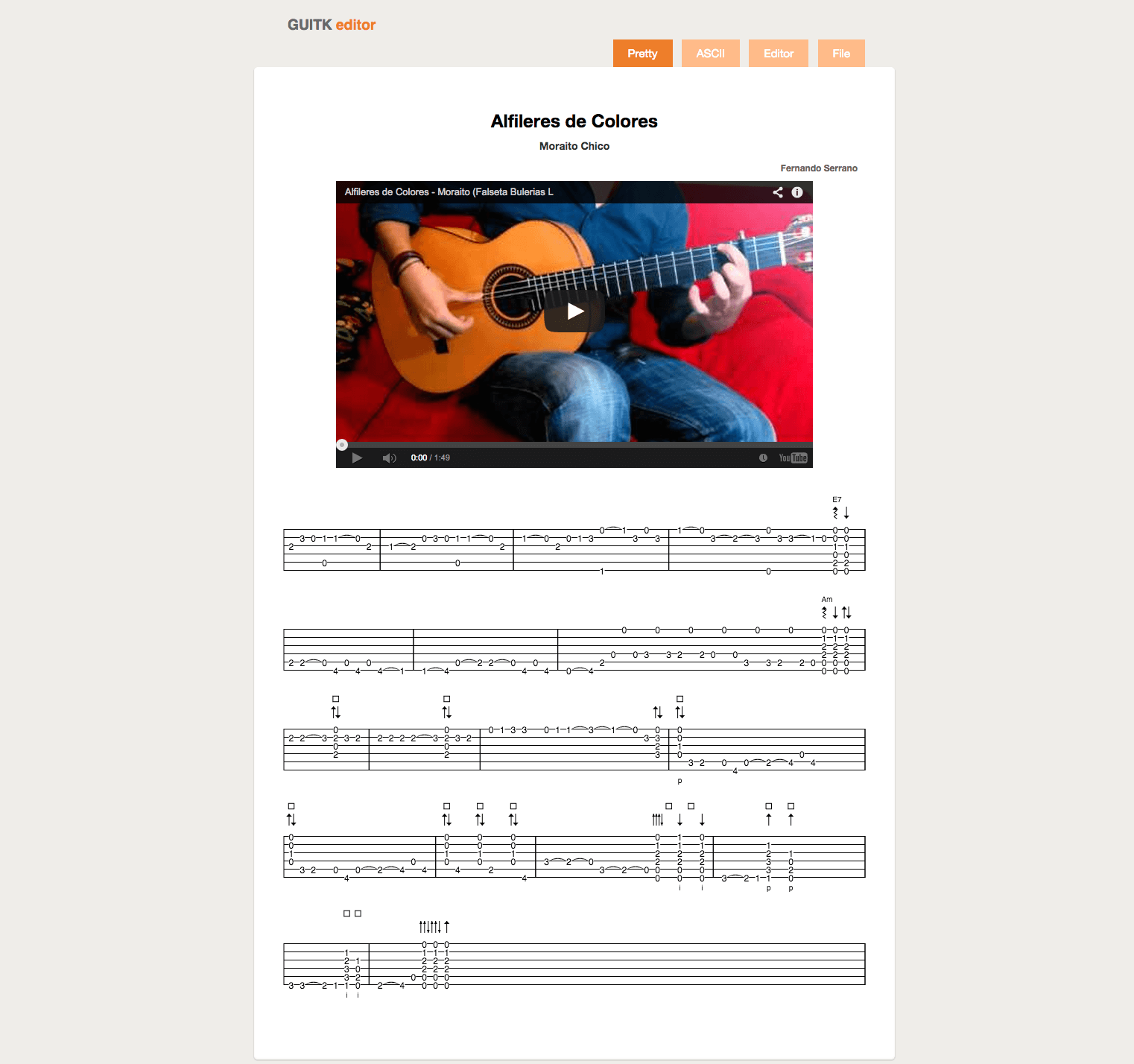
I’m so proud of the final result as it got everything I wanted in the beginning: easy to use (At least I think so :D ), it allows you to include text parts (for lyrics, comments, etc.), youtube links (In case you want to add some reference video) and tablature section.
The tablature section was made for speed in mind, so even if you can click for every option, there’s a simple key that will do the same action, so you’ll be faster using just the keyboard while transcripting.
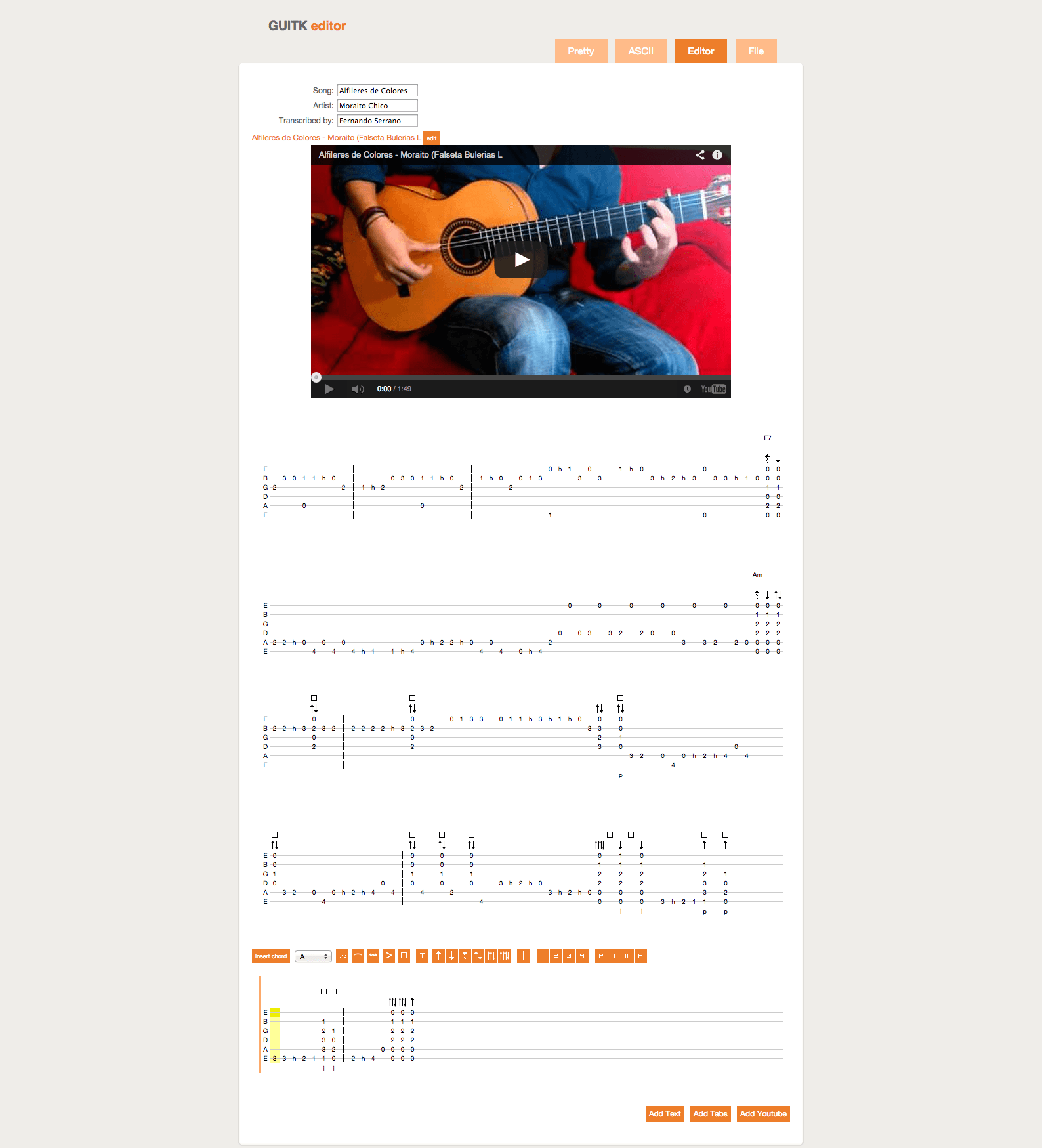
I created a canvas renderer to render the tablature in a pretty way more similar to the format you could see in music books.
Every tablature could be saved and loaded in a JSON file format.
I developed also an API to let third parties to include the editor in their webapps.
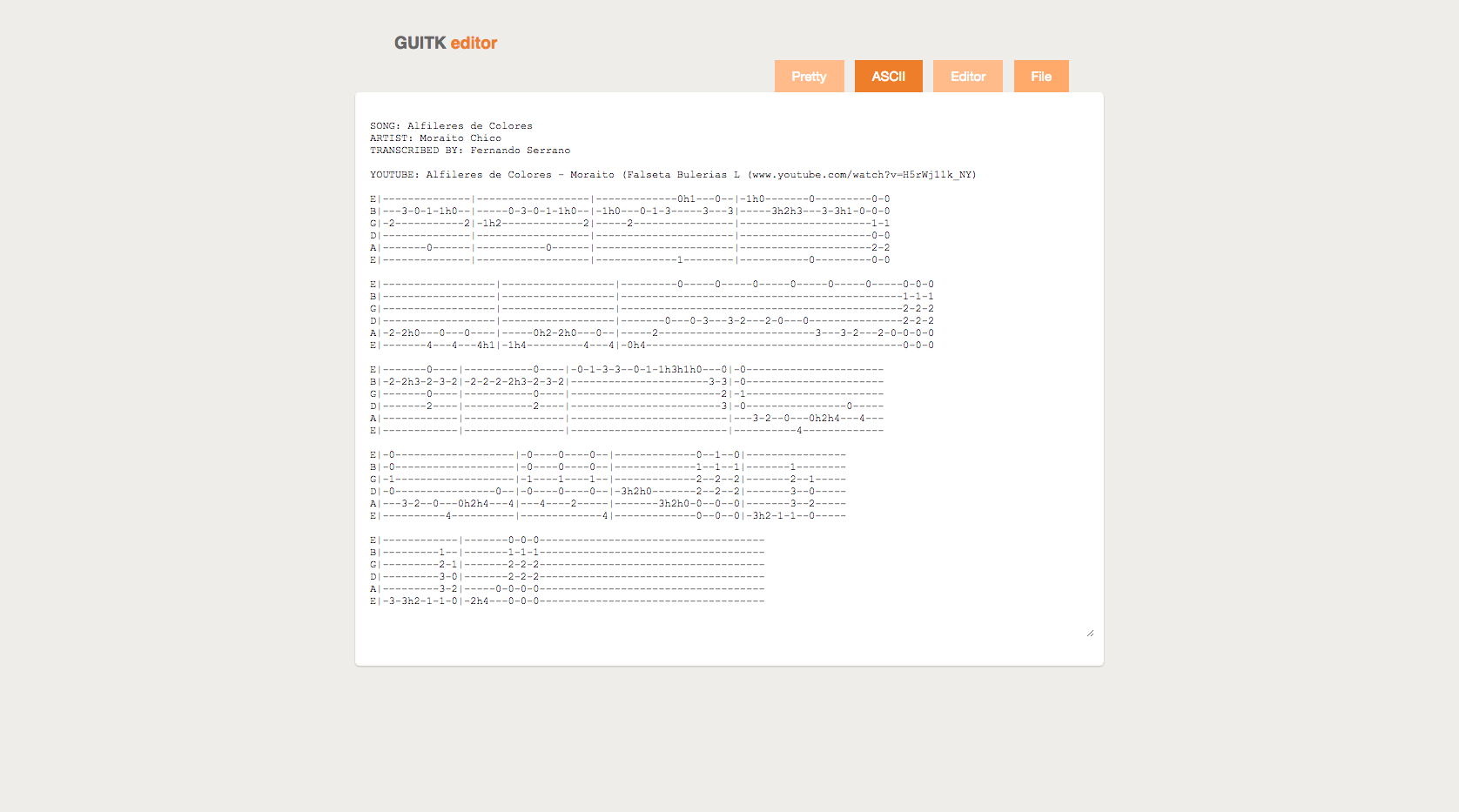
Main features
- Import/Export from custom JSON file format
- Two rendering modes:
- Traditional text ASCII
- "Pretty" print using HTML5 Canvas
- Many tablature symbols: hammen-on, pull-off, slide up/down, vibrato, rasgueado, etc.
- Include youtube videos, text or tabs sections
- Full of keyboard shortcuts
- Easy to integrate on your own webapp using an iframe
Tutorial
Example of use:
Shortcuts for TAB section
- cursors: Move cursor
- [0..9]: Insert fret number at cursor
- ins: Insert empty cell
- del: Delete current cell
- shift+ins: Insert empty column
- shift+del: Delete column
- s: Slide
- h: Hammer on/Pull off
- v: Vibrato
- g: Accents
- t: Add/Modify text layer
- p,m,i,a: Select right hand finger
- shift+[1,2,3,4]: Select left hand finger
API
You can easy integrate this editor in your website using an iframe:
<iframe
id="guitk-editor"
scrolling="no"
src="www.guitk.com/editor?api-mode=editor&show-song-info=false"
name="guitk-editor"
width="880"
height="500"
scrolling="auto"
frameborder="1">
</iframe>
Once you have the iframe working, you need to communicate with the editor using postMessage
and receiveMessage
:
- postMessage
- load-tabs: We will send the tablature in JSON format and the editor will load and show it.
- get-tabs: We ask for the current tablature in JSON format.
- receiveMesasge
- return-tabs: It's the answer from the editor to the
get-tabs
message - on-resize: It's called everytime that the editor height is modified, in case that we want to modify the iframe size too.
- return-tabs: It's the answer from the editor to the
Load tabs in editor
To load a tablature in the editor we just need to send the JSON structure using the load-tabs
message:
var win = document.getElementById( "guitk-editor" ).contentWindow;
win.postMessage( { action: "load-tabs", tabs: myTablatureInJSON }, "www.guitk.com/editor" );
It's a good practise to wait until the editor is already loaded before send this message, so we could wait until the editor ´´´onload´´´ event is triggered to send our message:
document.getElementById( "guitk-editor" ).onload= function() {
var win = document.getElementById( "guitk-editor" ).contentWindow;
win.postMessage( { action: "load-tabs", tabs: myTablatureInJSON }, "www.guitk.com/editor" );
};
Retrieve tabs from the editor
Once we're done editing a tablature we would like to save it in our application. So in that case we could query the editor to send us the tablature in JSON format to do whatever we want in our webapp.
To do this we should send a get-tabs
message:
First we define a callback that will receive the answer when we'll query the tabs:
window.addEventListener("message", receiveMessage, false);
function receiveMessage(event)
{
if (event.data.action=="return-tabs") {
var da a = JSON.parse( event.data.tabs );
}
}
Now we can ask for the tablature and wait for the answer to the previous callback:
win.postMessage({action:"get-tabs"}, "www.guitk.com/editor" );
Editor resize
Everytime the editor changes its height a message is sent to your web, so you could change the size of the iframe for example, or just ignored it.
We just need add a new handler to our receiveMessage
function:
function receiveMessage( event )
{
if ( event.data.action == "return-tabs" ) {
// This will contains the tablature data in json format
var data = event.data.tabs;
}
else if ( event.data.action == "on-resize" ) {
// event.data.height will return the size in pixels of the editor height
document.getElementById( "guitk-editor" ).height = ( 50 + event.data.height ) + "px";
}
}